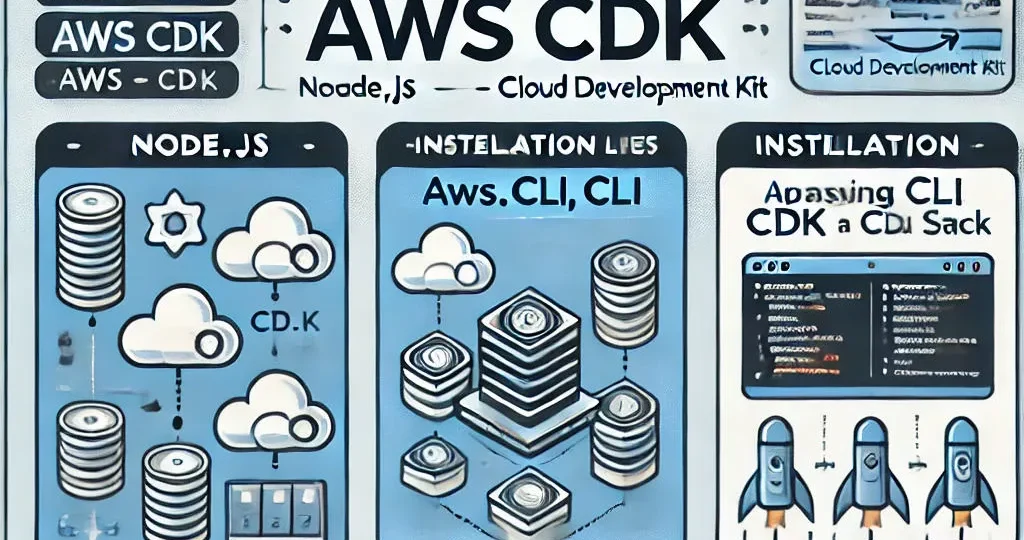
Detailed Tutorial
Setup AWS profile
The AWS Command Line Interface (CLI) is a powerful command-line tool allowing you to interact directly with AWS services from your terminal. The installation process may vary depending on your operating system.
# macOS brew install awscli # Windows wget https://awscli.amazonaws.com/AWSCLIV2.msi msiexec.exe /i https://awscli.amazonaws.com/AWSCLIV2.msi # Linux (Ubuntu) sudo apt install awscli
To be able to access your AWS account from the AWS CLI, you need to set up an AWS Profile. There are 2 ways you can do that:
Access and secret key from IAM User
AWS Single Sign-on (SSO) user
In this article, to be able to quickly set up an AWS Profile, we will choose method number 1.
- Log in to the AWS Console
- Go to IAM > Users

- Select IAM user, click Security credentials và
create access key
.

- Save access key và secret key
- Set up AWS profile for AWS CLI
aws configure
AWS Access Key ID [None]: <insert_access_key>
AWS Secret Access Key [None]: <insert_secret_key>
Default region name [None]: <insert_aws_region>
Default output format [json]: json
Your Information credentials will be saved at ~/.aws/credentials
with name profile [default], You can check by command
aws sts get-caller-identity
{
"UserId": "xxx",
"Account": "xxx",
"Arn": "arn:aws:iam::xxx:user/xxx"
}
Setup AWS CLI
We use the NPM package manager to install AWS CDK. This is a common installation method for all operating systems (MacOS, Windows, Linux,…). Run the following command on your terminal to install the AWS CDK toolkit
npm install -g aws-cdk
# Check version of AWS CDK
cdk version
# List commands of cdk
cdk --help
Create A Project
To initialize a new AWS CDK project, you can run the cdk init
command, you can customize your desired template and programming language.
cdk init --list
# output
Available templates:
* app: Template for a CDK Application
└─ cdk init app --language=[csharp|fsharp|go|java|javascript|python|typescript]
* lib: Template for a CDK Construct Library
└─ cdk init lib --language=typescript
* sample-app: Example CDK Application with some constructs
└─ cdk init sample-app --language=[csharp|fsharp|go|java|javascript|python|typescript]
This may be your first time using AWS CDK, so let’s start with sample-app. This project will help you understand how a stack is created on AWS Cloud using AWS CDK.
mkdir aws-cdk-sample-app-application
cd aws-cdk-sample-app
cdk init sample-app --language=python
The command will automatically generate code for the project using the structure below.
├── README.md
├── app.py
├── aws_cdk_sample_app_application
│ ├── __init__.py
│ └── aws_cdk_sample_app_stack.py
├── cdk.json
├── requirements-dev.txt
├── requirements.txt
├── source.bat
└── tests
├── __init__.py
└── unit
├── __init__.py
└── test_aws_cdk_sample_app_application_stack.py
Setup python env
python3 -m venv .venv
# Cài đặt các dependencies
pip install -r requirements.txt
pip install -r requirements-dev.txt
You can see in the aws_cdk_sample_app_application
folder there is a stack, that contains the code for creating an SQS queue and SNS topic + subscription. Since we are using AWS CDK with sample-app, these resources are already defined.
Build A Application
After we create a new AWS CDK project with a stack (aws_cdk_sample_app_stack_application.py
) that includes the resources configured in the sample app. To build the application, we use the cdk synth command. This command will synthesize the stack(s) defined in the AWS CDK application into a CloudFormation template.
cdk synth
output
Resources:
AwsCdkSampleApplicationQueueF7B3BB55:
Type: AWS::SQS::Queue
Properties:
VisibilityTimeout: 300
UpdateReplacePolicy: Delete
DeletionPolicy: Delete
Metadata:
aws:cdk:path: AwsCdkSampleApplicationStack/AwsCdkSampleApplicationQueue/Resource
AwsCdkSampleApplicationQueuePolicy2A67F8BD:
Type: AWS::SQS::QueuePolicy
Properties:
PolicyDocument:
Statement:
- Action: sqs:SendMessage
Condition:
ArnEquals:
aws:SourceArn:
Ref: AwsCdkSampleApplicationTopic10BF8361
Effect: Allow
Principal:
Service: sns.amazonaws.com
Resource:
Fn::GetAtt:
- AwsCdkSampleApplicationQueueF7B3BB55
- Arn
Version: "2012-10-17"
Queues:
- Ref: AwsCdkSampleApplicationQueueF7B3BB55
Metadata:
aws:cdk:path: AwsCdkSampleApplicationStack/AwsCdkSampleApplicationQueue/Policy/Resource
AwsCdkSampleApplicationQueueAwsCdkSampleApplicationStackAwsCdkSampleApplicationTopicF438882A8BACBD15:
Type: AWS::SNS::Subscription
Properties:
Endpoint:
Fn::GetAtt:
- AwsCdkSampleApplicationQueueF7B3BB55
- Arn
Protocol: sqs
TopicArn:
Ref: AwsCdkSampleApplicationTopic10BF8361
DependsOn:
- AwsCdkSampleApplicationQueuePolicy2A67F8BD
Metadata:
aws:cdk:path: AwsCdkSampleApplicationStack/AwsCdkSampleApplicationQueue/AwsCdkSampleApplicationStackAwsCdkSampleApplicationTopicF438882A/Resource
AwsCdkSampleApplicationTopic10BF8361:
Type: AWS::SNS::Topic
Metadata:
aws:cdk:path: AwsCdkSampleApplicationStack/AwsCdkSampleApplicationTopic/Resource
CDKMetadata:
Type: AWS::CDK::Metadata
Properties:
Analytics: v2:deflate64:H4sIAAAAAAAA/1WNQQ6DIBREz+Ief6UuegAvYLX7BpGmv1qwfIgxhLs3QNKkm5k3k0nmDPzSQlOJnWo5L/WKE4TRCbkwsdM90IcgXL3yinUPXSBrb1aUx68sMTLSBGH0E0mLm0Oj0+Iv38yGMrUZYkw4KDLeyvzRGT1jWkbWH+5p9KkF3gDn1YsQa+u1w7eCofgXw//H1sAAAAA=
Metadata:
aws:cdk:path: AwsCdkSampleApplicationStack/CDKMetadata/Default
Condition: CDKMetadataAvailable
Conditions:
CDKMetadataAvailable:
Fn::Or:
- Fn::Or:
- Fn::Equals:
- Ref: AWS::Region
- af-south-1
- Fn::Equals:
- Ref: AWS::Region
- ap-east-1
- Fn::Equals:
- Ref: AWS::Region
- ap-northeast-1
- Fn::Equals:
- Ref: AWS::Region
- ap-northeast-2
- Fn::Equals:
- Ref: AWS::Region
- ap-northeast-3
- Fn::Equals:
- Ref: AWS::Region
- ap-south-1
- Fn::Equals:
- Ref: AWS::Region
- ap-south-2
- Fn::Equals:
- Ref: AWS::Region
- ap-southeast-1
- Fn::Equals:
- Ref: AWS::Region
- ap-southeast-2
- Fn::Equals:
- Ref: AWS::Region
- ap-southeast-3
- Fn::Or:
- Fn::Equals:
- Ref: AWS::Region
- ap-southeast-4
- Fn::Equals:
- Ref: AWS::Region
- ca-central-1
- Fn::Equals:
- Ref: AWS::Region
- ca-west-1
- Fn::Equals:
- Ref: AWS::Region
- cn-north-1
- Fn::Equals:
- Ref: AWS::Region
- cn-northwest-1
- Fn::Equals:
- Ref: AWS::Region
- eu-central-1
- Fn::Equals:
- Ref: AWS::Region
- eu-central-2
- Fn::Equals:
- Ref: AWS::Region
- eu-north-1
- Fn::Equals:
- Ref: AWS::Region
- eu-south-1
- Fn::Equals:
- Ref: AWS::Region
- eu-south-2
- Fn::Or:
- Fn::Equals:
- Ref: AWS::Region
- eu-west-1
- Fn::Equals:
- Ref: AWS::Region
- eu-west-2
- Fn::Equals:
- Ref: AWS::Region
- eu-west-3
- Fn::Equals:
- Ref: AWS::Region
- il-central-1
- Fn::Equals:
- Ref: AWS::Region
- me-central-1
- Fn::Equals:
- Ref: AWS::Region
- me-south-1
- Fn::Equals:
- Ref: AWS::Region
- sa-east-1
- Fn::Equals:
- Ref: AWS::Region
- us-east-1
- Fn::Equals:
- Ref: AWS::Region
- us-east-2
- Fn::Equals:
- Ref: AWS::Region
- us-west-1
- Fn::Equals:
- Ref: AWS::Region
- us-west-2
Parameters:
BootstrapVersion:
Type: AWS::SSM::Parameter::Value<String>
Default: /cdk-bootstrap/hnb659fds/version
Description: Version of the CDK Bootstrap resources in this environment, automatically retrieved from SSM Parameter Store. [cdk:skip]
As you can see, the cdk synth
the command displays a CloudFormation template in your terminal. This template is also saved as JSON in the cdk.out
folder so it can be used when deploying to AWS.
Deploy A Application
To deploy the application after being synthesized by the cdk synth
command.
After you have finished installing, you can continue with deploying the AWS CDK application.
You need to bootstrap the AWS CDK application before you can deploy it to AWS2, this helps create the necessary resources for the CDK toolkit to deploy your application, including: S3 bucket, IAM roles, SSM Parameter,…
cdk bootstrap --profile htadev # replace your profile
cdk deploy --profile htadev
Output
⏳ Bootstrapping environment aws://xxx/ap-southeast-1...
Trusted accounts for deployment: (none)
Trusted accounts for lookup: (none)
Using default execution policy of 'arn:aws:iam::aws:policy/AdministratorAccess'. Pass '--cloudformation-execution-policies' to customize.
✅ Environment aws://xxx/ap-southeast-1 bootstrapped (no changes).

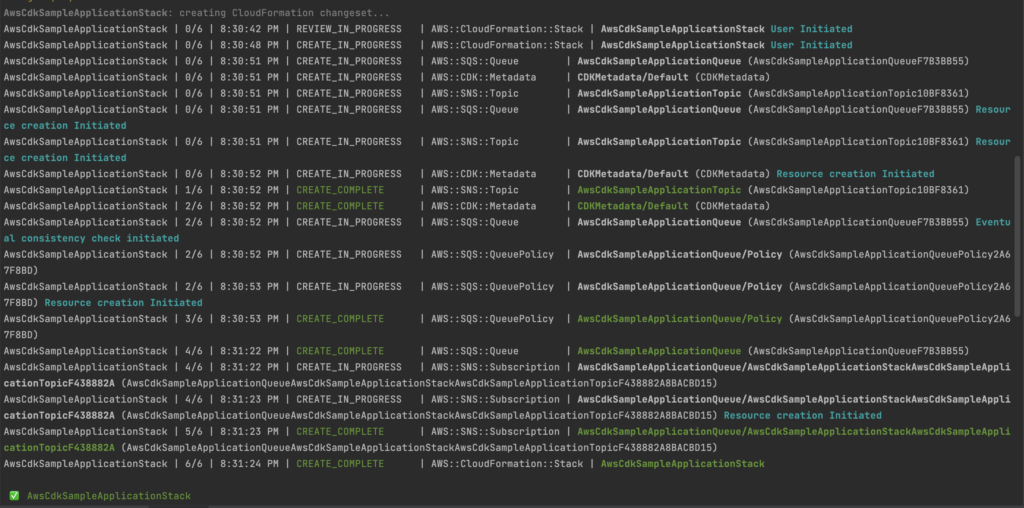
If you want to delete the newly created stack from your AWS account, run the following command
cdk destroy --profile htadev # replace your profile
Summary
Through this tutorial, you learned how to install AWS CDK, and set up and initialize an AWS CDK project.
Reference
https://docs.aws.amazon.com/cdk/v2/guide/getting_started.html
RELATED POSTS
View all